Virtual android phones
Try to create virtual android phones on server.
Ref.: Virtual Smartphone over IP
Some android emulators:
- Android-x86
http://www.android-x86.org/ - ARChon Custom Runtime
http://archon-runtime.github.io/ - Official Android SDK Emulator w/HAXM
http://developer.android.com/sdk/index.html - Android on Intel Architecture
https://01.org/android-ia - ARC Welder
https://developer.chrome.com/apps/getstarted_arc
Remote control
Linux Ubuntu 14.04 64bit
Prerequisite:
- Android-x86
- VirtualBox
- Python…
Steps
- download android-x86-5.1-rc1.iso
- dkpg -i virtualbox-5.0_5.0.12-104815-Ubuntu-trusty_amd64.deb
Need to enable Intel VT-X or AMD-V in your bios configuration - install android-x86 in virtualbox
- cotrol virtualbox on console
Start VM
If run as root:
- sudo -u jeff -H VBoxManage startvm Android
background mode - sudo -u jeff -H VBoxHeadless -s Android -v on -e “TCP/Ports=31000”
Stop VM - sudo -u jeff -H VBoxManage startvm Android
*
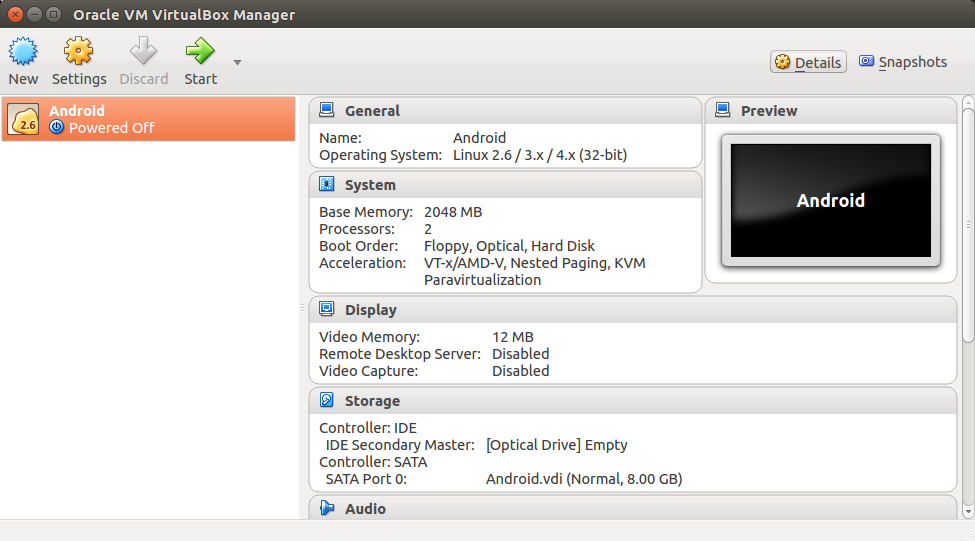
Test
TCP socket
In android-x86 press Alt+F1 entry console mode
netcfg
check ip
ifconfig eth0 xx.xx.xx.xx netmask 255.255.255.0
Alt+F7 GUI mode
1, Server send a message -> Android VM app receive the message
Server
client.py
# -*- coding: utf-8 -*-
import socket
def Main():
host = '192.168.42.142'
port = 10090
mySocket = socket.socket()
mySocket.connect((host,port))
message = input(" -> ")
while message != 'e':
mySocket.send(message.encode())
message = input(" -> ")
mySocket.close()
if __name__ == '__main__':
Main()
Android VM app
SockerService.java
package com.example.user.myapplication;
import android.app.IntentService;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
import android.widget.TextView;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.net.ServerSocket;
import java.net.Socket;
public class SocketService extends IntentService {
public static final String ACTION_MyIntentService = "com.example.user.myapplication.RESPONSE";
public static final String ACTION_MyUpdate = "com.example.user.myapplication.UPDATE";
public static final String EXTRA_KEY_IN = "EXTRA_IN";
public static final String EXTRA_KEY_OUT = "EXTRA_OUT";
public static final String EXTRA_KEY_UPDATE = "EXTRA_UPDATE";
private Context context;
private String name;
private int Port = 10090;
private ServerSocket server_socket;
private Socket socket;
private InputStream in;
private OutputStream out;
private BufferedReader reader;
private String messgae = null;
public SocketService() {
super("SocketService");
// TODO Auto-generated constructor stub
}
public SocketService(Context context, String name) {
super("SocketService");
this.context = context;
this.name = name;
}
protected void onHandleIntent(Intent intent) {
// TODO Auto-generated method stub
while (true) {
try {
socket = server_socket.accept();
in = socket.getInputStream();
reader = new BufferedReader(new InputStreamReader(in));
messgae = reader.readLine();
Intent intentResponse = new Intent();
intentResponse.setAction(ACTION_MyIntentService);
intentResponse.addCategory(Intent.CATEGORY_DEFAULT);
intentResponse.putExtra(EXTRA_KEY_OUT, messgae.toString());
sendBroadcast(intentResponse);
} catch (Exception e) {
e.printStackTrace();
Log.i("service exception", e.getMessage());
}
}
}
public void onCreate() {
// TODO Auto-generated method stub
super.onCreate();
try {
server_socket = new ServerSocket(Port);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void onDestroy() {
// TODO Auto-generated method stub
super.onDestroy();
}
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.user.myapplication" >
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme" >
<activity android:name=".MainActivity" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name=".SocketService" >
</service>
</application>
</manifest>
*
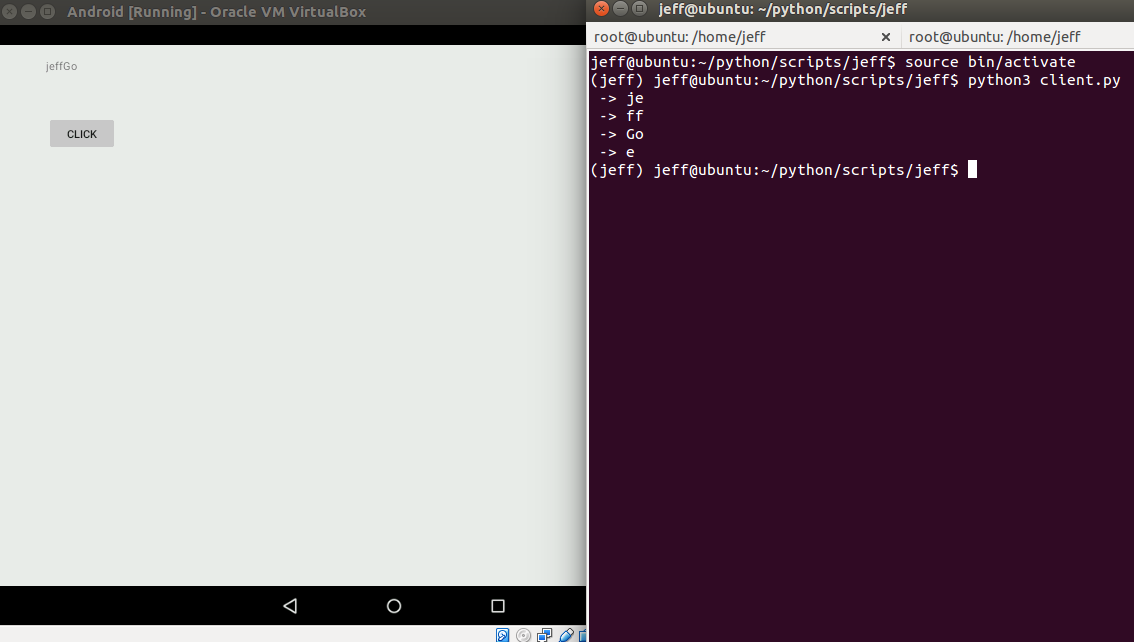
2, Android VM send a message once finishing satrt -> Server receive the message
~ TODO ~
Use simple-web-vnc
First, get the application window name.
$ xwininfo -root -tree | grep VirtualBox
0x4000001 “VirtualBox”: (“VirtualBox” “VirtualBox”) 10x10+10+10 +10+10
0x1a0000c “Oracle VM VirtualBox Manager”: (“VirtualBox” “VirtualBox”) 975x513+0+0 +765+196
0x420000c “Android [Running] - Oracle VM VirtualBox”: (“VirtualBox” “VirtualBox”) 800x622+0+0 +238+176
Modify code in Simple-web-vnc
server.go
func (conn *connection) appStreaming() {
windowName := "Android [Running] - Oracle VM VirtualBox"
wn := C.CString(windowName)
conn.appHandle = C.app_create(1024, 0,0, 800, 600, wn);
...
}
Notice: Mouse position mapping still has some problems between Android VM and canvas !!!
Demo